The Cloud Cost Fix Hidden in the Tools You Already Use
Cloud engineers are under constant pressure to optimize cloud costs—but the irony? Some of the best cost-saving features are already built into your CI/CD pipelines, Kubernetes clusters, and monitoring platforms. The real challenge isn’t finding new tools—it’s unlocking the underutilized, misconfigured, or overlooked capabilities in the ones you already have.
This guide cuts through the noise and shows you exactly how to activate cost-saving features in the tools you already have—with tactical steps, code snippets, and quick wins you can implement today.
If you want to:
- Stop overpaying for test environments that run long after they’re needed
- Automatically scale workloads based on actual usage—not guesswork
- Set up cost anomaly alerts before your finance team comes knocking
…then keep reading. This isn’t another vague best practices guide. You’ll walk away with real configurations, practical examples, and expert-level cost optimization tactics.
Let’s get started.
CI/CD pipelines: Optimize costs while shipping code faster
CI/CD pipelines are designed to streamline deployments, but they also introduce hidden costs if not properly managed. Test environments that never expire, orphaned cloud resources from failed builds, and oversized staging environments can quietly inflate cloud bills.
If your team relies on GitHub Actions, GitLab CI/CD, or Jenkins to automate deployments, there are built-in capabilities to curb cloud waste—you just need to enable them. Likewise, Terraform can help enforce cost constraints before resources spiral out of control. To start optimizing your CI/CD workflows, implement these best practices:
1. Set Auto-TTL for test environments in Terraform
Test environments are often spun up for temporary testing but rarely shut down on time. This results in unnecessary cloud spend that compounds over time. Terraform can tag resources with TTL (time-to-live) values, which can be enforced via scheduled cleanup jobs or Lambda functions. This ensures that infrastructure self-destructs after a set period, reducing manual cleanup efforts.
resource "aws_instance" "test_env" {
instance_type = "t3.micro"
tags = {
Name = "test-env"
Expiry = "24h"
}
}
Beyond just setting a TTL, you should ensure that your cloud provider supports automated cleanup. Some environments may require additional scripting or AWS Lambda functions to enforce deletions. Another effective approach is to schedule Lambda cleanup jobs that remove expired resources proactively. Additionally, using terraform apply -destroy
in automation workflows can help ensure that test environments are systematically torn down after testing.
2. Trigger cost visibility alerts in CI/CD pipelines
CI/CD pipelines are great for automating deployments, but without guardrails, they can deploy expensive resources without oversight. Setting up cost anomaly alerts within CI/CD workflows ensures that developers are aware of unexpected cloud spend before it escalates.
Here’s how to trigger cost anomaly alerts using AWS CloudWatch:
{
"AlarmName": "CI-CD-Cost-Spike",
"ComparisonOperator": "GreaterThanThreshold",
"Threshold": 500,
"MetricName": "EstimatedCharges",
"Namespace": "AWS/Billing",
"Period": 3600,
"EvaluationPeriods": 1
}
For GitLab CI/CD specifically, you can implement cost checks directly in your pipeline:
variables:
MAX_COST_THRESHOLD: "500"
stages:
- cost-check
- deploy
cost_estimation:
stage: cost-check
script:
- |
# Use AWS CLI to get cost forecast
FORECAST=$(aws ce get-cost-forecast \
--time-period Start=$(date +%Y-%m-%d),End=$(date -d "+30 days" +%Y-%m-%d) \
--metric UNBLENDED_COST \
--granularity MONTHLY \
--output json | jq -r '.Total.Amount')
# Ensure FORECAST variable is correctly parsed
FORECAST=${FORECAST:-0}
# Convert to floating point for comparison
if (( $(echo "$FORECAST > $MAX_COST_THRESHOLD" | bc -l) )); then
echo "Estimated cost ($FORECAST) exceeds threshold ($MAX_COST_THRESHOLD)"
exit 1
else
echo "Estimated cost ($FORECAST) is within the acceptable threshold."
fi
rules:
- if: $CI_PIPELINE_SOURCE == "merge_request_event"
3. Enforce cost constraints in Terraform
Infrastructure provisioning often leads to over-provisioned and costly instances if left unchecked. Terraform’s Sentinel policies can block high-cost resource types before deployment, preventing unnecessary expenses.
Here’s how to set a Terraform Sentinel policy that prevents provisioning of oversized instances:
policy "enforce-instance-size" {
rules = {
main = {
enforcement_level = "hard-mandatory"
condition = {
all aws_instance as instance {
instance.type not in ["m5.4xlarge", "r5.8xlarge"]
}
}
}
}
}
To enforce these cost constraints across teams, use Terraform Cloud’s Policy Sets, which apply governance at scale. You can further strengthen cost controls by combining these policies with AWS Service Control Policies (SCPs) to ensure compliance across cloud accounts. Regularly auditing Terraform state files also helps detect potential violations before they become costly mistakes.
How CloudBolt Helps
CloudBolt transforms episodic CI/CD cost controls into continuous, automated optimization through:
- Cloud Native Actions (CNA) that provide AI-driven cost analysis and automated remediation before deployment
- Seamless integration with Jenkins, GitLab, and GitHub Actions to enforce budget constraints through automated policy checks
- Machine learning actions that optimize infrastructure configurations based on historical usage patterns
- Automated policy enforcement that prevents non-compliant deployments while suggesting cost-effective alternatives
Kubernetes cost optimization: Mastering resource efficiency
Running workloads in Kubernetes (K8s) clusters—whether on AWS EKS, GCP GKE, or Azure AKS—can quickly escalate cloud costs if resources aren’t managed properly. Over-allocated CPU and memory, idle nodes, and limited cost visibility all contribute to unnecessary waste.
Thankfully, tools like Prometheus provide workload monitoring, while KEDA enables event-driven autoscaling, reducing unnecessary resource allocation. To fully leverage these tools, apply the following best practices:
1. Enable Horizontal Pod Autoscaling (HPA) and KEDA for smarter scaling
Many teams overprovision Kubernetes resources to avoid performance bottlenecks, which leads to wasted cloud spend. The better approach? Let workloads scale dynamically based on real-time demand using Horizontal Pod Autoscaling (HPA) and KEDA.
apiVersion: autoscaling/v2
kind: HorizontalPodAutoscaler
metadata:
name: my-app-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: my-app
minReplicas: 2
maxReplicas: 10
metrics:
- type: Resource
resource:
name: cpu
target:
type: Utilization
averageUtilization: 75
For more advanced scaling based on custom metrics, use KEDA:
apiVersion: autoscaling/v2
kind: HorizontalPodAutoscaler
metadata:
name: my-app-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: my-app
minReplicas: 2
maxReplicas: 10
metrics:
- type: Resource
resource:
name: cpu
target:
type: Utilization
averageUtilization: 75
2. Use Prometheus to identify over-provisioned resources
Prometheus is a powerful tool for monitoring Kubernetes workloads, but many teams fail to set up alerts for inefficient resource allocation. Without proper visibility, over-provisioned CPU and memory requests can silently drive up cloud costs.
To detect underutilized resources, configure Prometheus alerts that trigger when CPU or memory utilization stays below a certain threshold for an extended period:
apiVersion: monitoring.coreos.com/v1
kind: PrometheusRule
metadata:
name: underutilized-resources
spec:
groups:
- name: resource-alerts
rules:
- alert: HighCPURequestsLowUsage
expr: |
sum(rate(container_cpu_usage_seconds_total{namespace="production"}[5m]))
/ sum(kube_pod_container_resource_requests{namespace="production", resource="cpu"}) < 0.2
for: 10m
labels:
severity: warning
annotations:
summary: "High CPU requests with low actual usage"
description: "Pods in the production namespace are requesting too much CPU but using less than 20% for 10 minutes."
3. Enable Cluster Autoscaler for intelligent scaling
Kubernetes nodes that sit idle waste money without adding any performance benefits. The Cluster Autoscaler dynamically adjusts node counts based on workload demand, ensuring that teams aren’t paying for unnecessary capacity.
Here’s how to configure Cluster Autoscaler on AWS EKS:
apiVersion: apps/v1
kind: Deployment
metadata:
name: cluster-autoscaler
spec:
template:
spec:
containers:
- name: cluster-autoscaler
image: k8s.gcr.io/autoscaling/cluster-autoscaler:v1.22.1
command:
- ./cluster-autoscaler
- --cloud-provider=aws
- --nodes=1:10:my-node-group
- --scale-down-utilization-threshold=0.5
How CloudBolt Helps
CloudBolt enhances Kubernetes cost optimization through its Augmented FinOps capabilities:
- AI-driven workload analysis that predicts resource needs with documented average savings of 50% through intelligent rightsizing
- Automated pod scheduling decisions that balance cost optimization with performance requirements
- Real-time detection and termination of idle resources across multiple clusters through Cloud Native Actions
- Unified cost governance across hybrid and multi-cloud Kubernetes environments through the CloudBolt Agent
Monitoring & cost alerting: Stop cost surprises before they happen
Cloud cost overruns often happen due to a lack of real-time cost visibility. Engineers don’t always have clear insights into which resources are driving up spend, leading to wasted budget allocation. Without proactive monitoring, unexpected cost spikes can go unnoticed until they become expensive problems.
If you’re using AWS CloudWatch, Azure Monitor, Google Cloud Operations, or Datadog, these platforms have powerful built-in cost monitoring and alerting capabilities that can help mitigate waste before it escalates. To proactively monitor cloud costs and prevent unnecessary spending, apply these best practices:
1. Set up cost anomaly detection in CloudWatch and Azure Monitor
One of the biggest challenges in cloud cost management is identifying sudden spikes before they impact the budget. Instead of waiting for a shocking bill, engineers can set up cost anomaly detection to trigger real-time alerts when spend exceeds expected thresholds.
Here’s how to set up comprehensive cost monitoring across multiple services using Terraform:
apiVersion: apps/v1
kind: Deployment
metadata:
name: cluster-autoscaler
spec:
template:
spec:
containers:
- name: cluster-autoscaler
image: k8s.gcr.io/autoscaling/cluster-autoscaler:v1.22.1
command:
- ./cluster-autoscaler
- --cloud-provider=aws
- --nodes=1:10:my-node-group
- --scale-down-utilization-threshold=0.5
2. Tag resources for cost allocation visibility
Many cloud environments suffer from poor cost visibility due to missing resource tagging. Without a proper tagging strategy, teams struggle to allocate costs accurately, making it difficult to identify which services, teams, or projects are driving expenses.
Applying standardized cost ownership tags in AWS, Azure, and GCP ensures that every cloud resource has a designated owner and purpose. A basic tagging structure could look like this:
resource "aws_instance" "app_server" {
tags = {
"Environment" = "Production"
"Owner" = "DevOps Team"
"CostCenter" = "CloudOps-Budget"
}
}
Beyond just applying cost allocation tags, organizations should enforce mandatory tagging policies using AWS Organizations Service Control Policies (SCPs) or Azure Policy to maintain consistency across environments. Regular audits and automated cleanup scripts help detect untagged or misclassified resources before they impact cost tracking. Additionally, hierarchical tagging structures—such as tagging by project, department, or team—improve cost accountability and reporting.
3. Use Datadog custom dashboards for cost visibility
Datadog provides deep observability into cloud costs, but many teams don’t take advantage of its cost dashboards to visualize and track spending trends in real-time. Custom dashboards allow engineers to correlate cost spikes with resource usage patterns.
For example, a Datadog dashboard can be configured to display:
- Underutilized resources that should be right-sized or decommissioned.
- Cost trends per application, service, or team to drive accountability.
- Real-time alerts for cloud cost spikes, allowing engineers to react before a budget is blown.
To get the most value from Datadog, teams should configure custom cost visibility dashboards that highlight cost trends and underutilized resources in real time. This can be done by:
- Creating a new dashboard and selecting “Cost & Usage” as the data source.
- Setting up custom widgets to track spending by service, team, and environment, providing granular visibility into cost distribution.
- Defining threshold alerts that notify teams when sudden cost increases occur, allowing for proactive adjustments before budgets are exceeded.
How CloudBolt Helps
CloudBolt transforms basic monitoring into proactive cost management through its Augmented FinOps platform:
- Unified dashboard showing cost metrics across AWS, Azure, GCP, and private cloud environments
- Cloud Native Actions that automatically remediate cost anomalies before they impact budgets
- Machine learning algorithms that reduce insight-to-action time from weeks to minutes
- Integration with existing monitoring tools to provide a single source of truth for cloud costs
How to take it further: Scaling cost optimization with automation
At this point, you’ve optimized cost-saving features in the tools you already use—but cost efficiency isn’t a one-time fix. The real challenge is keeping costs under control as environments scale, teams grow, and workloads shift.
That’s where automation changes the game. Instead of reacting to cost spikes after they happen, CloudBolt helps teams move from cost monitoring to cost prevention. By embedding intelligent automation across environments, you can:
- Eliminate manual cleanup: Stop chasing down orphaned resources and let automation handle it.
- Ensure every dollar spent aligns with usage: No more guesswork in resource allocation.
- Scale cloud governance effortlessly: Implement guardrails that adapt in real time.
Instead of simply alerting you to a problem, CloudBolt helps you solve it before it starts—transforming cost optimization from a reactive process into a fully automated, AI-driven strategy.
You’ve seen what’s possible with built-in cost controls—now see how automation takes it further. Get a demo.
Related Blogs
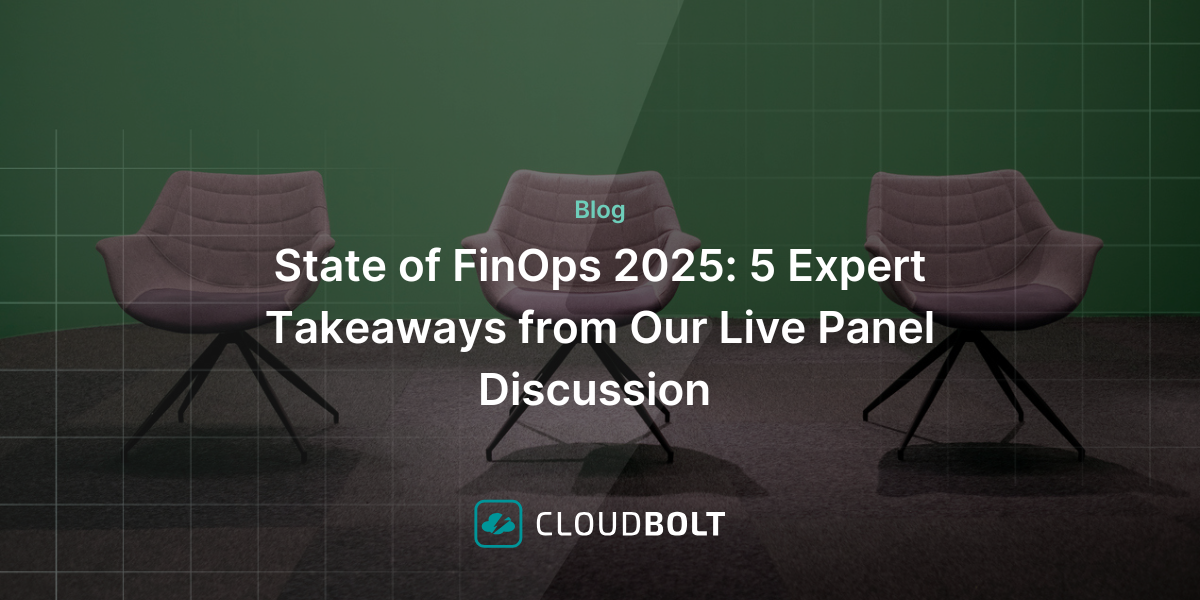
State of FinOps 2025: 5 Expert Takeaways from Our Live Panel Discussion
The landscape of FinOps is shifting fast. AI-driven cost management, governance at scale, and the expanding scope of responsibilities are…